import { SafeAreaView, StyleSheet, SectionList, Text, View } from 'react-native';
import {sectionsData} from './data.js'
import { Dimensions } from 'react-native';
const { width, height } = Dimensions.get('window');
export default class App extends React.Component {
renderSectionHeader=({menuSection})=>{
return (
<Text style={styles.text}>{String(menuSection).toUpperCase()}</Text>
)
}
renderItem=({data})=>{
return (
<View style={styles.view}>
<View style={styles.view1}>
<Text style={styles.text1}>{data.name}</Text>
<Text style={styles.text2}>{data.price}</Text>
</View>
<Text></Text>
</View>
)
}
render() {
return <SafeAreaView style={styles.container}>
<SectionList
sections={sectionsData}
keyExtractor={item => item.uid}
renderSectionHeader={({ section: menuSection }) => this.renderSectionHeader(menuSection)}
renderItem={({ item: data }) =>this.renderItem({data})}
></SectionList>
</SafeAreaView>
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
},
text: {
paddingTop: 30,
paddingHorizontal: 10,
fontSize: 30,
fontWeight: 'bold',
color: '#E3771C'
},
view:{
marginVertical: 10,
paddingHorizontal: 30
},
view1:{
flexDirection: 'row',
justifyContent: 'space-between'
},
text1:{
paddingRight: 20,
fontWeight: 'bold',
fontSize: 20,
width: width*0.7
},
text2:{
fontSize: 20
}
});
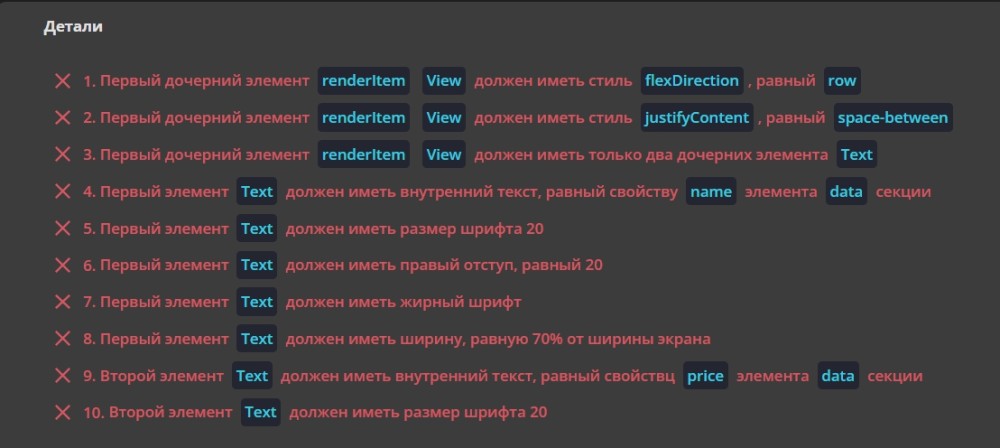